Create a braided stent
- Peter Mortier
- Jan 19
- 2 min read
Updated: 2 days ago
In this example, we will show you how to create a braided stent. Braided stents are innovative medical devices designed to support blood vessels and maintain their patency. These stents are constructed using a series of interwoven strands of material, which provides flexibility and adaptability to the vessel's movement.
Creating a 3D model of a braided stent can be challenging. However, with the robust Python scripting capabilities offered by HelloTriangle, you can generate a fully parametric 3D model using minimal code. This advanced example is best investigated by visualizing and printing all intermediate steps of the script. At the bottom, you can also see some alternative designs which were created by simply changing of the input parameters.
Please check our post on script-based parametric modeling for some general information about this topic.
from hellotriangle import shapes
import numpy as np
# input parameters
D = 5.0 # average stent diameter
d = 0.12 # wire diameter
ds = 0.001 # distance between crossing wires
ny = 10 # number of wires in 1 direction
l = 10.0 # approximate length of the stent
a = 60.0 # pitch or wire angle
ne = 4 # number of elements in a wire between 2 crossings
# determine vertical (dy) and horizontal (dx) dimension of single unit
dy = 3.14*D/ny
dx = dy/np.tan(a*np.pi/180.0)
# determine number of horizontal repititions on a single unit
nx = int(round(l/dx))
# create single wire unit
wire = shapes.line([-dx/4.0, 0.0, 0.0],[dx/4.0, 0.0, 0.0],2*ne)
wire.coords[:,2] = (1 - (wire.coords[:,0]/(dx/4.0))**2)*(d+ds)/2.0
# compose half of base unit
wire1a = wire.shear(1, 0, dy/dx)
# sweep circle around centerline
cir = shapes.circle(8).scale(d/2.0)
wire1a = cir.sweep(wire1a, normal = 2)
# complete base unit
wire1b = wire1a.reflect(2).translate([dx/2.0, dy/2.0, 0.0])
wire1 = wire1a + wire1b
# create wires in other direction
wire2 = wire1.reflect(2).reflect(1)
# replicate
wireCyl = (wire1+wire2).replicateXYZ(n=[nx, ny, 1],step=[dx, dy, 1.0])
# coordinate transformation (from cylindrical coordinates to cartesian)
tube = wireCyl.translate([0.0, 0.0, D/2.0]).cylindrical(dir = (2,1,0), scale = (1.0, 360.0/(3.14*D), 1.0))
# draw
draw(tube, color = "grey")
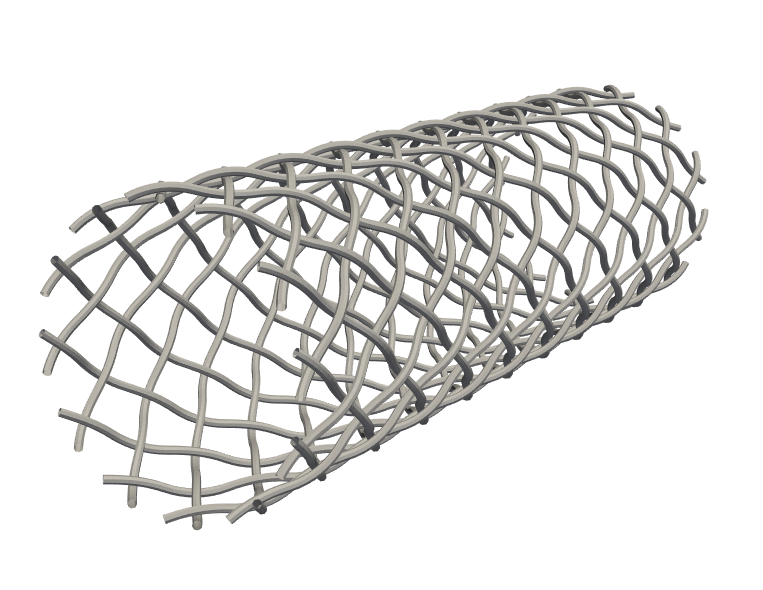

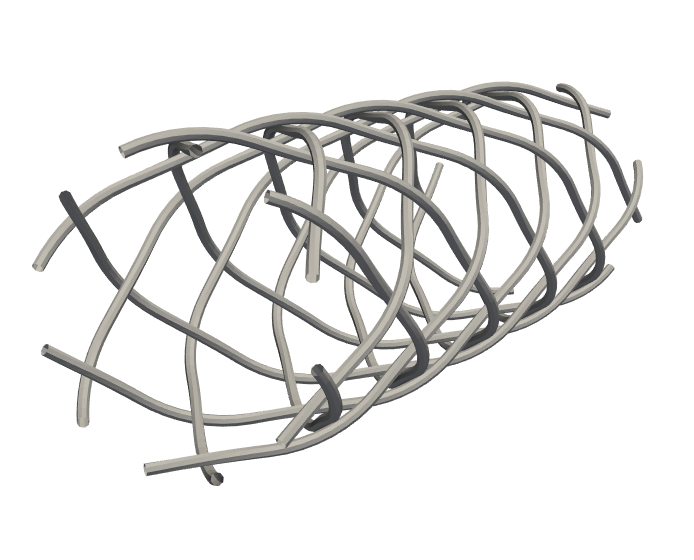