Convert spherical to cartesian coordinates
- Peter Mortier
- Mar 11
- 1 min read
Updated: 1 day ago
Coordinate transformations play a crucial role in various fields, and can also be very powerful for 3D modeling. One common transformation is to convert spherical to Cartesian coordinates. Spherical coordinates, defined by radius, polar angle, and azimuthal angle, are ideal for representing spherical objects (hence the name). By creating 3D models in spherical coordinates, and then converting to Cartesian coordinates, designers can create advanced 3D models, as illustrated in this example.
This example is best investigated by visualizing intermediate steps of the script.
If you are interested in coordinate transformations, you may also want to take a look at our example showing how to convert cylindrical to cartesian coordinates.
from hellotriangle import shapes
import numpy as np
# create rectangular grid in XY plane consisting of triangles
base = shapes.quad(eltype='tri3').subdivide(500)
# add sinus wave to Z coordinates along X-axis
base.coords[:,2] = np.sin(base.coords[:,0]*2*np.pi*10)
# shear the mesh in the X-direction (dir=0), based on the Y coordinate (dir=1)
base = base.shear(0, 1, 0.5)
# scale so that X values range between 0-360 (degrees), and Y values range between 0-180 (degrees)
base = base.scale([360.0, 180.0, 1.0])
# translate so that Y values range between -90 and 90 (degrees), and that average Z value (= radius) is 15.0
base = base.translate([0.0, -90.0, 15.0])
# convert from spherical to cartesian coordinates
sphere = base.spherical()
# draw
draw(sphere, color = "#2BB786")
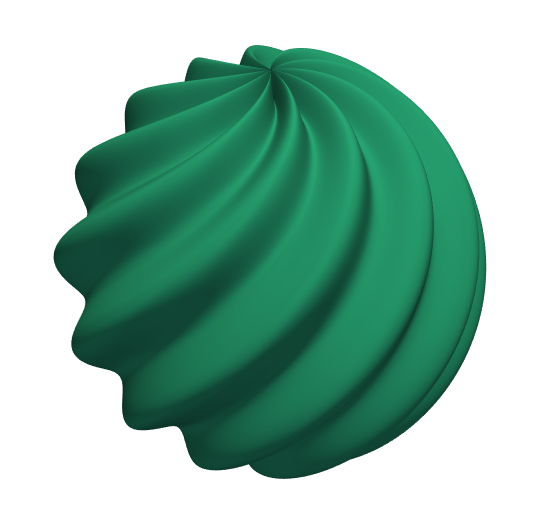