Why use Python for 3D modeling
In this post, we’ll explore why Python 3D modeling is a compelling alternative to traditional GUI-based tools, particularly for engineers and scientists.
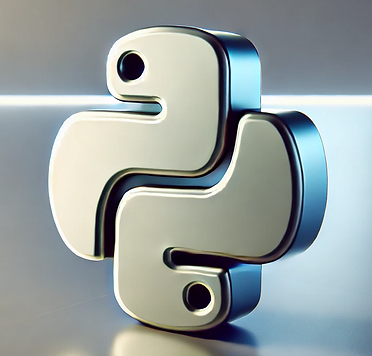
What Is Python 3D Modeling?
3D modeling plays a crucial role in fields like 3D printing, computer-aided design (CAD), and engineering simulations. However, traditional GUI-based tools often lack efficiency when handling repetitive tasks. This is where Python for 3D modeling comes in—allowing users to automate complex operations, improve accuracy, and enhance reproducibility.
In this article, we will:
Explain mesh-based 3D modeling and its importance.
Highlight limitations of GUI-based 3D modeling software.
Discuss the advantages of Python 3D modeling and show how it simplifies complex tasks.
What Is Mesh-Based 3D Modeling?
Mesh-based 3D models are digital representations of objects, constructed from elements that define their geometry. These models can represent surfaces (surface meshes) or entire volumes (volume meshes) and play a vital role in numerous technical applications.
Surface Models:
Represent the external shape of an object.
Composed of vertices, edges, and faces, typically in the form of triangles or quadrilaterals.
Common file format: .stl
Used for 3D printing and reconstructing real-world objects, such as organs.
If you want to learn more about editing triangulated surfaces, check our post on online stl editing
Volume Meshes:
Describe both the interior and exterior of an object.
Composed of volumetric elements like tetrahedrons or hexahedrons.
Essential for structural simulations (Finite Element Analysis - FEA) and fluid simulations (Computational Fluid Dynamics - CFD).
Mesh-based 3D modeling is crucial in scientific and engineering fields for simulating stress and strain in materials, modeling fluid flow through complex geometries, and analyzing 3D structures. These models deliver precise representations of complex shapes, and allow for efficient rendering and detailed visualization.
While graphical user interface (GUI) software dominates the 3D modeling space, it often lacks efficiency for repetitive or advanced tasks. Let’s explore an example to illustrate this further.
Limitations of GUI-Based 3D Modeling
GUIs provide easy access to 3D modeling, allowing users to intuitively click on menus and buttons, explore features, and swiftly grasp the basics. However, for repetitive or complex tasks, GUIs soon become inefficient, and there are certain challenges that cannot be addressed through a GUI.
Imagine you need to analyze 100 segmented human bones (e.g., femurs) from medical CT image. Your goal is to compute the minimum cross-sectional area for each of the 100 bones. Using GUI-based software, this process would involve:
Importing each bone model.
Manually orienting the bones.
Taking multiple cross-sectional measurements along the axis.
Repeating these steps for all 100 bones.
This approach is not only time-consuming but also prone to errors, and there’s no guarantee that measurements are accurate or reproducible. Additionally, how can you ensure that you performed a measurement at the location with the smallest cross-section?
Now, let's take a look why the use of Python-based 3D modeling can be a very powerful and efficient alternative.
Why choose Python for 3D modeling?
Python has become the most popular programming language among engineers and scientists for a good reason. Let’s explore how Python 3D modeling outperforms traditional software.
Automation
Python excels at automating repetitive tasks. If you need to apply the same process to hundreds of models, a Python script can save significant time by performing the task programmatically. For instance, extracting specific geometric properties can be automated with just a few lines of code.
Reproducibility
Unlike GUI-based tools, where the steps taken may be forgotten or poorly documented, When using Python-based 3D modeling, every process is scripted, ensuring precise and repeatable results.. You can always refer back to your code to understand and replicate the exact process. This is particularly valuable in research settings, where reproducibility is a cornerstone of credibility and collaboration.
Powerful problem-solving
Some tasks are nearly impossible to perform manually in GUI tools. For example, finding the minimum cross-sectional area along the axis of a triangulated surface model requires numerous precise measurements. Python simplifies this with programmatic operations, ensuring both accuracy and efficiency.
Versatility
Python allows you to write custom functions to handle specific tasks, if some functionality would not be available. This flexibility is invaluable in technical fields where unique requirements are very common. From creating parametric models to performing advanced analyses, Python’s capabilities are virtually limitless.
Solving the Bone Analysis Problem with Python
Let’s revisit the problem of analyzing 100 STL files of human femur bones. Using Python, this seemingly daunting task becomes straightforward. Below is an outline of how this can be achieved using HelloTriangle:
Step 1: Import STL file
# import bone stl file
bone = Mesh.read('femur.stl')
draw(bone, color='grey')
In this situation, the bone is already aligned with the Z-axis. If it were not, we could use Python to calculate the principal axes and align the bone with the Z-axis.

Step 2: Slice the bone
As a second step, we will slice the model at regular intervals and compute the cross-sectional area for each slice. Slicing is performed by intersecting the model with planes perpendicular to the Z-axis.
# slice bone along its axis
slices = bone.slice(dir = [0.0 , 0.0 , 1.0], nplanes = 50)
draw(slices, color='#2BB786', line_width = 3.0)

Step 3: Find the minimal cross-sectional area
Finally, we need to determine the minimum cross-sectional area. In order to do this, a short function was written to calculate the area of a closed polygon. This nicely illustrates the flexibility of Python-based 3D modeling and shows how functionality can be easily added when it is not available. Information about the implemented formula can be found here.
def polygonArea(coordinates):
""" Calculate area of a closed polygon
Input is an array with x,y coordinates
Example input: [[0.0 , 0.0] , [1.0 , 0.0] , [1.0 , 1.0]]
"""
area = 0
ncoords = len(coordinates)
x = coordinates[:,0]
y = coordinates[:,1]
for i in range(ncoords):
if i < (ncoords - 1):
area += (x[i] * y[i+1] - x[i+1] * y[i]) / 2.0
else:
area += (x[i] * y[0] - x[0] * y[i]) / 2.0
return abs(area)
Now, let's compute the area of each slice. Our new function, "polygonArea," presumes that the list of coordinates is ordered. This means that consecutive coordinates in the array should represent adjacent points in the polygon. While the elements in our connectivity array (i.e., slice.elems) are ordered, the coordinates (slice.coords) are not. Thus, we must first obtain the ordered coordinates.
# calculate cross-sectional areas of each slice
areas = np.zeros(50)
for i , slice in enumerate(slices):
coords = slice.coords
orderedCoords = coords[slice.elems[:,0]]
areas[i] = polygonArea(orderedCoords)
# detect minimum area in central part of the bone
min = areas[10:-10].min()
# determine index of slice with minimal area
minSlice = np.where(areas == min)[0][0]
# highlight slice with minimal area
draw(slices[minSlice], color='red', line_width = 7.0)

This bone analysis example nicely illustrates the advantages of Python 3D modeling.
Conclusion
Python is revolutionizing 3D modeling by offering automation, accuracy, and flexibility beyond traditional GUI-based tools. Whether you’re working in engineering, 3D printing, or scientific research, Python 3D modeling allows for faster workflows and more precise results.
👉 Ready to explore Python for 3D modeling? Sign up for HelloTriangle today.